In this blog I am trying to put the things together a very basic level tutorial on understanding databases and more specifically how to implement one in MS SQL. Since the purpose of any database is to store data lets first look at the data itself. Say for example my name “Jayanth Kurup”. This is a piece of information I want to have saved somewhere on a computer so that I can access it at will; either directly or via website or some other piece of software like MS Excel etc.
Let’s look more closely at the data we have here. Jayanth Kurup is obviously a name but we need to now store it in a database before we do that we need to understand what kind of name it is. Is it a Full Name, Nick Name, User Name, Product Name, Preferred name etc. In this case it’s my Full Name. I would define this piece of information as
Full Name = Jayanth Kurup
It becomes pretty obvious that the data is the full Name for a person called Jayanth Kurup. Now we need to set some rules around what properties a Full name has. E.g a full Name will almost always contain only alphabets. However there may be a case for numbers to be listed here as well say Mark 1. So let’s assume for now that both alphabets and numbers are allowed. We can agree that a name will usually not exceed 64 characters in most countries and even if it does that’s usually an edge case. A key thing to remember is that we can’t account for every possible scenario and therefore need to leave a little space for flexibility but at the same time we can’t leave things open ended as that will allow all kinds of bad data to creep in. At this point we need to understand that not everybody has names that are the same length so it makes sense for us to store the data in a fashion such that it occupies just enough space to store the information.
Full Name = Jayanth Kurup (Accepted values = Alpha numeric, variable character length between 0 and 64)
The next point we need to address is: should we ever allow a scenario where a Full Name can be blank. i.e. Full Name = Nothing.
It might not seem like a big deal but it’s important to understand that certain types of data must always have a value assigned with it. For example an address will always have a zip code associated with it. Similarly everybody has a name so it’s unlikely we will ever have a scenario where the Full Name is NULL (NULL = nothing in database parlance).
Full Name = Jayanth Kurup (Accepted values = Alpha numeric, variable character length=1- 64, cannot be empty)
Naturally the next question we have to address is what language the data is stored in. e.g. my Name can be written in English as shown above or even in Hindi. So we need to define the language that this piece of information uses, in this case English.
Full Name = Jayanth Kurup (Accepted values = Alpha numeric, variable character length=1- 64, cannot be empty, Locale = English)
Its looks like we have some basic guidelines about what the information should look like before it can be assigned to the group call Full Name. However there is one more important area we need to address. In what context is this full Name being used? For example is this the full name of people in my address book, or the full name stored in an online website for their customer’s registration page. In the former it might make more sense for me to call it Contact Full Name and in the later it makes more sense to call it Customer Full Name. Other examples could be employee full name etc. Let’s assume this is a contacts database for now.
Contacts Full Name = Jayanth Kurup (Accepted values = Alpha numeric, variable character length=1- 64, cannot be empty, Locale = English)
Now it’s times to store this information in a database. Before we do this let’s look at how we would store this data in a MS Excel spread sheet.
We would naturally add a column called Contacts Full Name and then just below it enter the Value Jayanth Kurup. As shown below
Let’s make some comparisons between Excel and a database now.
MS Excel ~ MS SQL Server ~ Software that stores data
MS Excel Workbook ~ MS SQL Server Database ~ a container in which data related to a specific activity is stored e.g. contacts database, customer database.
MS Excel Workbook Spreadsheet ~ MS SQL Server Database Table ~ a container within the database that stores data of a very specific nature (an object here can mean a person, an address, a product catalogue etc.)
With this understanding you will see that storing data in a database is similar to storing data in a excel sheet. Please keep in mind the traditional database is far more powerful than Excel and implements a lot of check and balances to ensure to integrity and programmability of the data. Inserting a row into the excel sheet has many parallels with how you would insert a row in the database table. You would first need a workbook that you can access. Within the workbook you would access a specific sheet based on the type of data your storing e.g. you wouldn’t store the contact information such as email id in a sheet called hobbies.
With these basics in place let’s create our first database example, before we actually open up SQL Server and start creating the database and tables lets write down the scripts:
Create database ContactsDB — This statement creates a database in MS SQL Server
GO — This statement tells SQL Server to move on to the next statement
Create Table Contacts — This line is the starting line for the create table command. Unlike Excel where you can create the sheet first and define columns later, in SQL you need to do both together
( ContactsFullName Varchar (64) NOT NULL ) — The definition of the column which is equal to (Accepted values = Alpha numeric, variable character length=1- 64, cannot be empty, Locale = English)
GO
The above scripts will create a database and a table with the column ContactsFullName. In the next post we explore how to connect to MS SQL Server and execute the above code.
The code in Green needs to be executed together.
Please Consider Subscribing
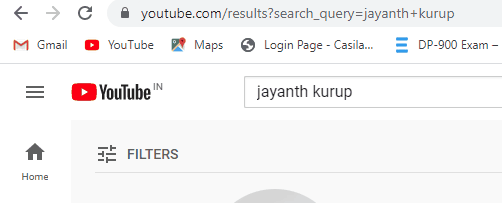